Picture
Time Limit: 2000MS | Memory Limit: 10000K | |
Total Submissions: 10229 | Accepted: 5421 |
Description
A number of rectangular posters, photographs and other pictures of the same shape are pasted on a wall. Their sides are all vertical or horizontal. Each rectangle can be partially or totally covered by the others. The length of the boundary of the union of all rectangles is called the perimeter.
Write a program to calculate the perimeter. An example with 7 rectangles is shown in Figure 1.
The corresponding boundary is the whole set of line segments drawn in Figure 2.
The vertices of all rectangles have integer coordinates.
Write a program to calculate the perimeter. An example with 7 rectangles is shown in Figure 1.
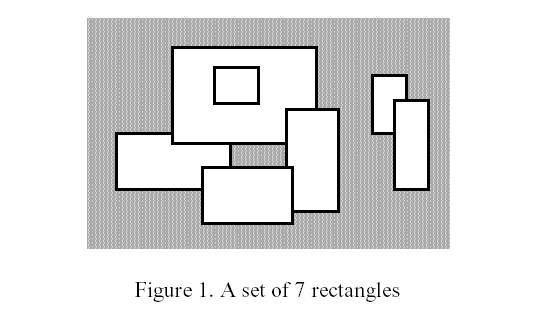
The corresponding boundary is the whole set of line segments drawn in Figure 2.
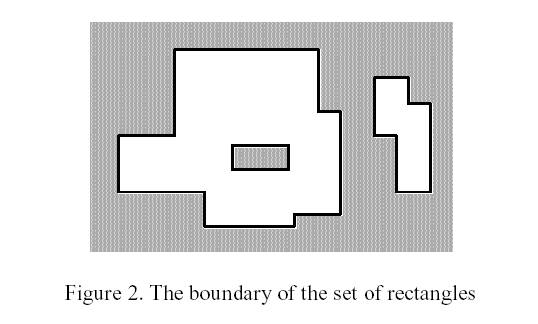
The vertices of all rectangles have integer coordinates.
Input
Your program is to read from standard input. The first line contains the number of rectangles pasted on the wall. In each of the subsequent lines, one can find the integer coordinates of the lower left vertex and the upper right vertex of each rectangle. The values of those coordinates are given as ordered pairs consisting of an x-coordinate followed by a y-coordinate.
0 <= number of rectangles < 5000
All coordinates are in the range [-10000,10000] and any existing rectangle has a positive area.
0 <= number of rectangles < 5000
All coordinates are in the range [-10000,10000] and any existing rectangle has a positive area.
Output
Your program is to write to standard output. The output must contain a single line with a non-negative integer which corresponds to the perimeter for the input rectangles.
Sample Input
7 -15 0 5 10 -5 8 20 25 15 -4 24 14 0 -6 16 4 2 15 10 22 30 10 36 20 34 0 40 16
Sample Output
228
题意:
给出 N(0 ~ 5000)个矩形,后给出每个矩形的左下坐标和右上坐标。所有的矩形给出之后,会有一个覆盖的轮廓,求出这个轮廓的周长。
思路:
线段树 + 扫描线 + 离散化。lc 维护左端点是否被覆盖,rc 维护右端点是否被覆盖,len 维护覆盖的长度,num 代表一共覆盖的顶点数, cover 代表覆盖区间的次数。将一个矩形的左竖边标记为 1,右竖边标记为 -1,注意算横边的时候,是用原来的 num 覆盖顶点数来算。之后再插入新的纵边,再查询总长 len 后,res +=( len - 上一次的 len)。注意更新 len 的时候,是更新离散化前的长度值。标记要用 += 值来标记,而不能直接用 1 或者 -1 来替换区间,因为可能会有一个区间被覆盖了两次的可能,比如样例:
2
5 1 10 2
6 1 15 2
如果直接用 1 或者 -1 覆盖得出的答案是 12,若用 += 的话则才是正确答案 22。
观察 push_up 函数:
void push_up (int node, int l, int r) { if (cover[node]) { len[node] = yy[r] - yy[l]; lc[node] = rc[node] = 1; num[node] = 2; //代表这个区间被覆盖 } else if (r - l == 1) { len[node] = lc[node] = rc[node] = num[node] = 0; //这个区间不被覆盖,且又是叶子节点 } else { len[node] = len[node << 1] + len[node << 1 | 1]; lc[node] = lc[node << 1]; rc[node] = rc[node << 1 | 1]; num[node] = num[node << 1] + num[node << 1 | 1]; if (rc[node << 1] && lc[node << 1 | 1]) num[node] -= 2; //这个区间不被覆盖,且不是叶子节点 } }
AC:
#include <cstdio> #include <cstring> #include <algorithm> #include <cmath> using namespace std; const int MAX = 20005; typedef struct { int x, y1, y2, temp; } node; node line[5005 * 2]; int yy[MAX]; int lc[MAX * 3], rc[MAX * 3], cover[MAX * 3]; int len[MAX * 3], num[MAX * 3]; bool cmp (node a, node b) { if (a.x != b.x) return a.x < b.x; return a.temp > b.temp; } void push_up (int node, int l, int r) { if (cover[node]) { len[node] = yy[r] - yy[l]; lc[node] = rc[node] = 1; num[node] = 2; } else if (r - l == 1) { len[node] = lc[node] = rc[node] = num[node] = 0; } else { len[node] = len[node << 1] + len[node << 1 | 1]; lc[node] = lc[node << 1]; rc[node] = rc[node << 1 | 1]; num[node] = num[node << 1] + num[node << 1 | 1]; if (rc[node << 1] && lc[node << 1 | 1]) num[node] -= 2; } } void build (int node, int l, int r) { if (r - l == 1) { cover[node] = 0; lc[node] = rc[node] = len[node] = num[node] = 0; } else { int mid = (r + l) >> 1; build(node << 1, l, mid); build(node << 1 | 1, mid, r); push_up(node, l, r); cover[node] = 0; } } void updata (int node, int l, int r, int cl, int cr, int c) { if (cl > r || cr < l) return; if (cl <= l && cr >= r) { cover[node] += c; push_up(node, l, r); return; } if (r - l == 1) return; int mid = (r + l) >> 1; updata(node << 1, l, mid, cl, cr, c); updata(node << 1 | 1, mid, r, cl, cr, c); push_up(node, l, r); } int main () { int t; while (~scanf("%d", &t)) { int n = 0, ans = 0; while (t--) { int x1, y1, x2, y2; scanf("%d%d%d%d", &x1, &y1, &x2, &y2); line[n].x = x1; line[n].y1 = y1; line[n].y2 = y2; line[n++].temp = 1; line[n].x = x2; line[n].y1 = y1; line[n].y2 = y2; line[n++].temp = -1; yy[ans++] = y1; yy[ans++] = y2; } sort(line, line + n, cmp); sort(yy, yy + ans); ans = unique(yy, yy + ans) - yy; build(1, 0, ans - 1); int res = 0, last = 0; for (int i = 0; i < n; ++i) { int cl = lower_bound(yy, yy + ans, line[i].y1) - yy; int cr = lower_bound(yy, yy + ans, line[i].y2) - yy; if(i) res += num[1] * (line[i].x - line[i - 1].x); updata(1, 0, ans - 1, cl, cr, line[i].temp); res += abs(len[1] - last); last = len[1]; } printf("%d\n", res); } return 0; }
相关推荐
这是我昨天刚实现的,之前在csdn上找了很多资料,但没找到在picture控件中绘制鼠标坐标和移动的,我在集结了他们的后实现了这个,其中还有我的txt文档,集结了很多网上博客论坛里的重要相关注意事项。和我一样的菜鸟...
该对话框是我学习MFC时老师给我布置的第一个任务,个人认为对于新手具有一定的参考价值,其中主要是熟悉各种常用控件的使用(包含八种控件)、定时器的使用以及在Picture Control中动态画正弦曲线。
iOS9 画中画 Picture in Picture
将opencv中的Mat格式的图片显示在mfc中的picture控件上,该程序已经被放在了一个函数中间,只需调用该函数ShowMatImgToWnd(CWnd* pWnd, cv::Mat img)就可以将所需的图片显示在picture控件上了,其中CWnd* pWnd参数中...
FANUC Picture User Manual
This sample demonstrates basic usage of Picture-in-Picture mode for handheld devices. The sample plays a video. The video keeps on playing when the app is turned in to Picture-in-Picture mode. On ...
通过程序空控制扫描仪完成图片的扫描,并将图片存入数据库中。
Halcon10.0+MFC编程 实现获取图片大小自适应PictureControl和存储,用户可以选取电脑里的任意图片,在获取之后还可以实现对图片进行任意位置的存储功能
微型集线器 具有Microsoft Style的GitHub主题。 Alexey 的推文的想法( ) 一键式从Google Chrome网上应用店安装 :down_arrow: :down_arrow: :down_arrow:
基于MFC对话框程序,利用picture控件显示位图,并可实现图像重绘。内有代码与实现之详细步骤。 附带WORD说明,比较适合初学者入门
一台日本米克隆无心磨FANUC PICTURE 二次开发例子,供大家学习。
一个在MFC对话框中显示OpenGL局部窗口的方法,通过Picture静态控件完成。 该代码在VC2005下编译完成,由于相对简单,完全可以适用VC6\VC2008等
动态向Picture Control控件中添加 .bmp .jpg .gif三种格式的图片
基于MFC对话框的OpenGL三维显示(picture control 类版)基于MFC对话框的OpenGL三维显示(picture control 类版)基于MFC对话框的OpenGL三维显示(picture control 类版)基于MFC对话框的OpenGL三维显示(picture control ...
在vc中 用picture控件 显示图片
X-Picture Converter是X-Picture家族软件的一员.X-Picture Converter是用来转换,压缩当前最流行的各种图形,图象格式的软件.您可以用很短的时间将要转换格式的图片快速处理完成.X-Picture Converter简洁的界面,简单的...
MFC点击Picture控件内,响应鼠标点击事件,并将点坐标显示在EDIT控件内。
VC PICTURE控件的使用,如何加载背景图片2009年04月19日 星期日 15:02vc picture控件的分类总结: (一) 非动态显示图片(即图片先通过资源管理器载入,有一个固定ID) (二) 动态载入图片(即只需要在程序中指定图片的...
Microsoft Office Picture Manager是一个基本的图片管理软件,是Microsoft Office中的一个组件(与一个基本版的Google的Picasa或Adobe的Photoshop Elements相似
mfc在picture控件的中心画两条分割线::::::::::::::